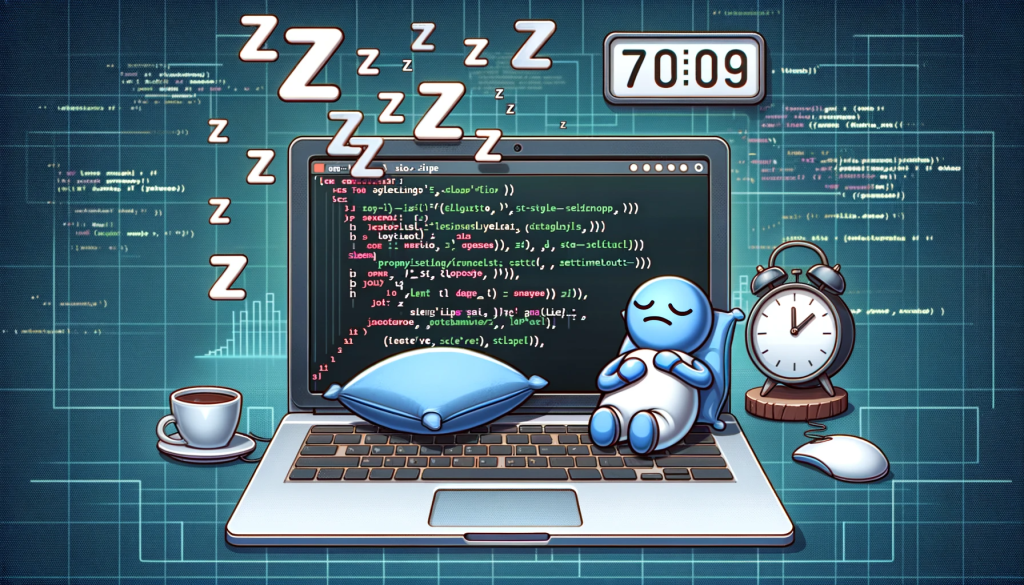
In many programming languages, a sleep()
function allows you to pause the execution of code for a set amount of time. JavaScript, however, does not have a native sleep()
function. This article explores how to implement a sleep-like functionality in JavaScript using Promises and the async/await
syntax.
Implementing Sleep in JavaScript
1. Using Promises to Create a Sleep Function
JavaScript allows you to create a sleep-like function using Promises. Here’s a simple implementation:
function sleep(ms) { return new Promise(resolve => setTimeout(resolve, ms)); }
With this function (javascript sleep promise
, sleep function javascript
), you can pause your code execution for a specified number of milliseconds.
2. Using the Sleep Function with Async/Await
To use this sleep function in an asynchronous context (javascript await sleep
, async sleep javascript
), you can combine it with the async/await
syntax:
async function run() { console.log('Hello'); await sleep(2000); // Sleep for 2 seconds console.log('World'); } run();
In this example (javascript sleep 2 seconds
), the await sleep(2000)
line causes a 2-second pause before executing the next line.
3. Sleep in JavaScript for Non-Asynchronous Code
For non-async functions (sleep javascript function
, sleep in javascript
), you can use the sleep function in a .then()
chain:
sleep(1000).then(() => { // Execute after 1 second console.log('Done sleeping'); });
4. Node.js Specific Sleep
In Node.js environments (nodejs sleep await
, node js sleep await
), the same approach using Promises works perfectly, providing a way to pause execution in server-side JavaScript.
Additional Considerations
-
- Non-Blocking Nature: JavaScript’s sleep implementation is non-blocking (
javascript async wait
,javascript blocking wait
), meaning it doesn’t halt the entire program but only pauses the execution within the async function.
- Non-Blocking Nature: JavaScript’s sleep implementation is non-blocking (
-
- Usage in Loops: Be careful when using
sleep()
inside loops (js sleep for 1 second
), as it can make asynchronous code more complex.
- Usage in Loops: Be careful when using
-
- Error Handling: Always handle potential errors when using Promises and async/await syntax.
-
- Use Cases: This sleep function is useful in various scenarios, like throttling requests (
javascript await delay
) or simulating network delays in development.
- Use Cases: This sleep function is useful in various scenarios, like throttling requests (
Conclusion
While JavaScript does not have a native sleep()
function, creating a custom sleep function using Promises and async/await syntax is straightforward and versatile. It allows developers to introduce delays in their code (how to sleep in javascript
, await sleep javascript
) effectively and in line with JavaScript’s asynchronous nature.